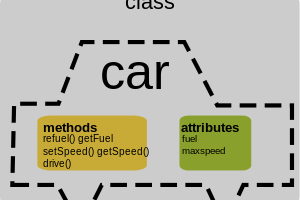
The final type of data structure that we will look at is the Data Transfer Object (DTO).
What’s a DTO?
Like the name says, the main purpose of a DTO is to transfer data. In it’s purest form, a DTO is a data structure that has only public properties, and no methods of any kind.
When are DTO’s used?
There are times when different parts of a system, need to communicate. Usually one part needs to ask the other part to do something and get a value in return. This is where DTOs shine. All of the data that is required either to make the request or to give the answer is packaged into a DTO before it’s sent.
One very common example of how DTOs are used is web APIs. When a request is done to a web api, usually the request is put in a DTO that is serialized and sent as JSON to the server, the server’s answer is also sent as JSON and then is deserialized into a DTO on the client side.
Benefits of using DTOs
One benefit of doing this is that it’s explicitly stated that there is a relationship between all the values stored in the DTO, and the name gives a clear indication of what the data is representing.
Another benefit is the encapsulation that DTOs provide. Different layers of a system, or even different systems can be written in totally different programming languages, and as long as they agree on the operations (and DTOs) that they will use, everything should work withouth problems.