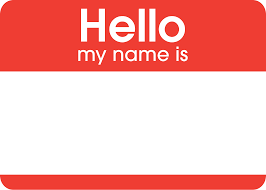
If we don’t give enough thought to the names we use, our code can become more difficult to understand because it’s unclear who does what.
Different variables that seem to be the same
In some cases, our code can be hard to understand because we don’t make meaningful distinctions when choosing names, take the following variables:
var customer; var customerInfo; var customerData;
It is unclear as to which data each variable holds. What’s the difference between them?
Unclear parameter names
Other times, the problem occurs when choosing the names for the parameters in our functions. Perhaps the clearest case for this would be simple mathematical operations.
int Multiply(int a, int b); int Divide(int a, int b);
For multiplication this might not be a significant problem since the order of the factors doesn’t affect the product. For the division, however, the result (quotient) will be different depending on which number is the one being divided (dividend) and which one is the divisor. Using better names the functions would look like this:
int Multiply(int multiplicand, int multiplier); int Divide(int dividend, int divisor);
While this might seem absurd (and maybe even confusing if you don’t remember the formal operand names) the code is very clear about the order in which it expects the parameters.
A “real world” example
Imagine a function in a financial system that takes funds from one account and deposits them in another. Which function prototype is clearer?
void TransferFunds(string a1, string a2, decimal d);
void TransferFunds(string originAccount, string destinationAccount, decimal amount);
Small improvements add up
Small details like this can make your code easier to understand. Code that is easy to understand is easier to modify, requires less comments and is less prone to have bugs. Any thing you can do to improve your code is worth doing.