Clean Code
Functions – The DRY Principle
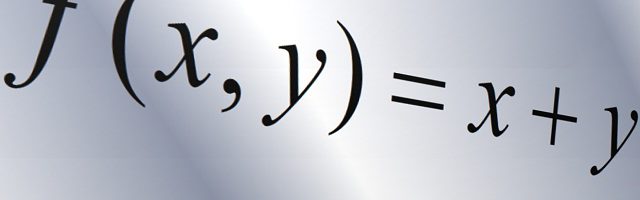
A very important lesson that every programmer has to learn at some point, is that duplication is bad. This is what the DRY Principle addresses. What is duplication? Simply put, duplication is having lines of code in your application, that are repeated in multiple places. Whenever you copy and paste a snippet of code, you…
Functions – Exceptions Instead Of Error Codes
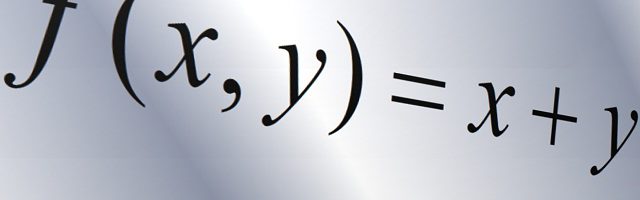
Maybe at some point you’ve written a function that had several possible results, and depending on the result, you had to do different things or show different error messages. One thing you might be tempted to do is have the function return a number representing the result code, or maybe the function returned an enumeration….
Functions – Command Query Separation
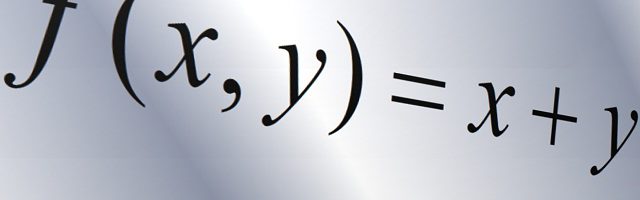
The idea of Command Query Separation is simple. You should have functions that perform actions that change the state of your system, and you should have functions that return (query) the current state of some part of the system, but your functions shouldn’t do both. That means you should have Commands and Queries, but should…
Functions – Side Effects
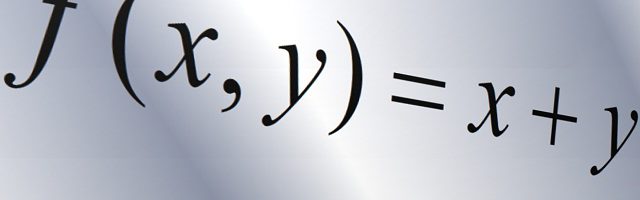
What is a side effect? According to Wikipedia, a function has a side effect when “it has on observable interaction with calling functions or the outside world”. Uncle Bob says that side effects are lies. A side effect happens when a function is supposed to do something, but it also does other things you don’t…
Functions – Arguments
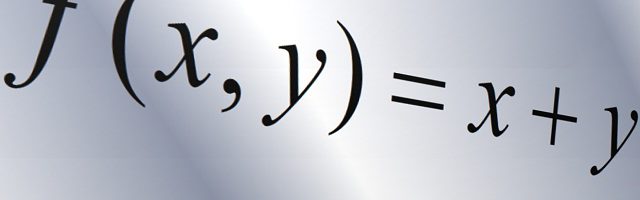
A very important thing you should have in mind when writing functions, is how many arguments each functions should have. Just like shorter functions are easier to understand, functions with few arguments are easier to understand and use. How many arguments should a function have? The short answer for this question is that a function…
Functions – Signs that your functions are doing more than one thing
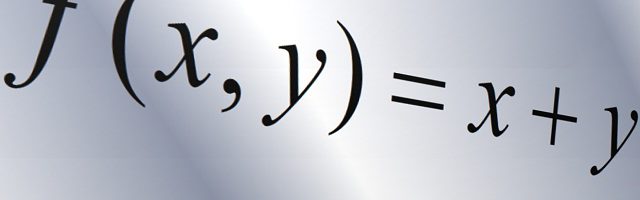
We’ve established that the key to having small functions is to make sure that they do one thing. A guideline to follow is that functions should remain on a single abstraction level. Now we will mention two very easy to detect signs that our functions may be doing more than one thing. Lots of indentation…
Functions – Levels of Abstraction
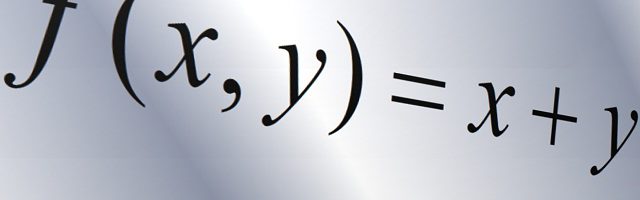
In the previous post, we talked about the importance of functions doing one thing. We briefly touched on something called abstraction levels. One of the simplest ways to be sure that your functions only do one thing, is to make sure that they stay on one abstraction level. Let’s try and explain this using a…
Functions – Smaller Is Better
Meaningful Names – Other Tips
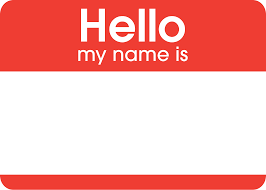
As a finish to the meaningful names part of our journey to produce clean code here are some other general tips mentioned in the book. Use Pronounceable Names Use names that you can pronounce. If you’ve followed the previous guidelines given, then most of your names should be clear and easy to say. Talking to…